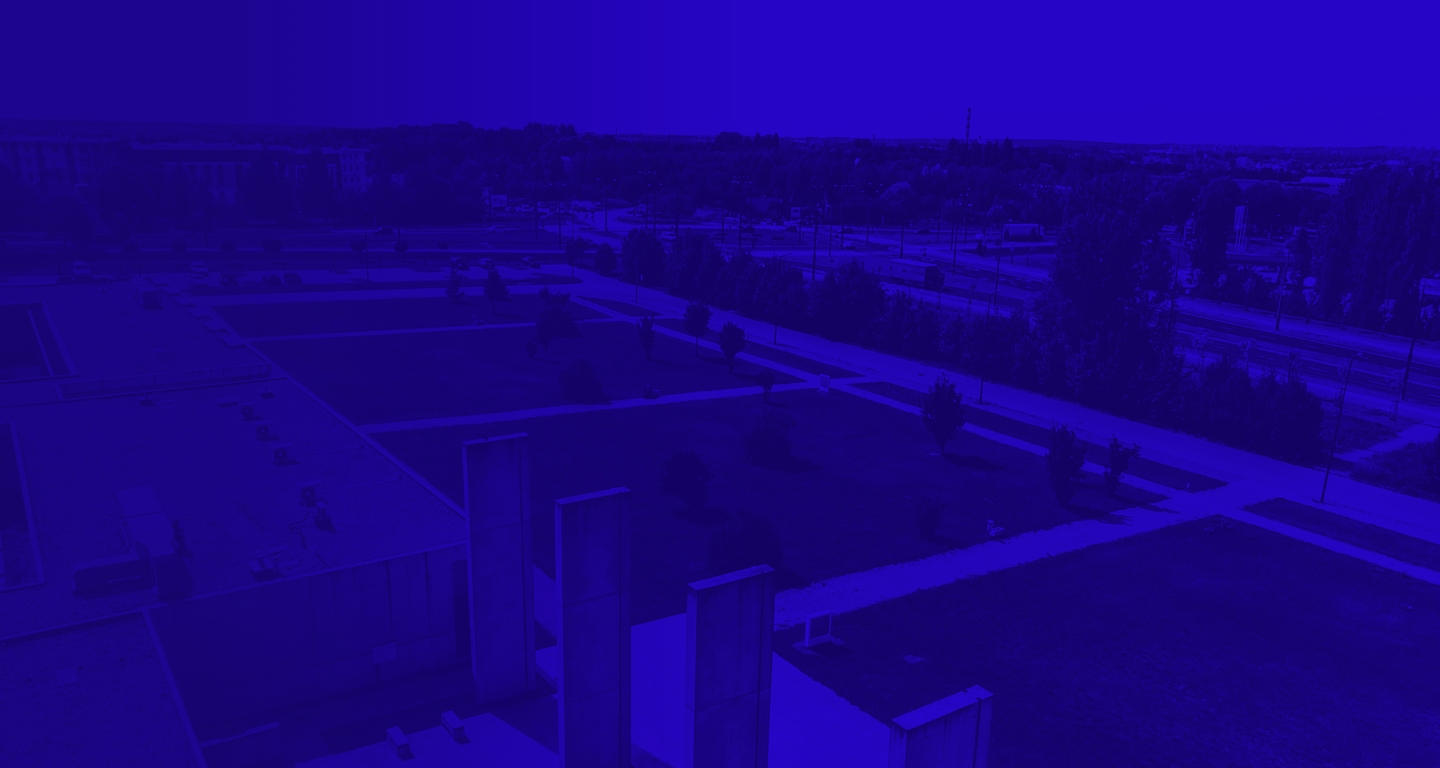
By default, regardless of whether the user does not exist, we have entered the wrong password or is blocked, the return message on the website based on FOSUserBundle (Symfony) always looks the same:
{
"code": 401,
"message": "Bad credentials"
}
You can easily get the exact message what the error has occurred, just register a simple subscriber which will enter the message from the first exception that occurred in the response (The first exception probably comes from FosUser and contains the first user validation error that contains the exact error message)
<?php
namespace App\Event\Subscriber;
use Lexik\Bundle\JWTAuthenticationBundle\Event\AuthenticationFailureEvent;
use Lexik\Bundle\JWTAuthenticationBundle\Events;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
class AuthenticationFailureSubscriber implements EventSubscriberInterface {
public static function getSubscribedEvents()
{
return [
Events::AUTHENTICATION_FAILURE => 'onAuthenticationFailure'
];
}
public function onAuthenticationFailure(AuthenticationFailureEvent $event)
{
$firstEvent = $event->getException();
while($firstEvent->getPrevious()) {
$firstEvent = $firstEvent->getPrevious();
}
$response = $event->getResponse();
$response->setMessage($firstEvent->getMessage());
$event->setResponse($response);
}
}
Thanks to this, we get phrases like:
{
"code": 401,
"message": "The presented password is invalid."
}
{
"code": 401,
"message": "User account is disabled."
}
{
"code": 401,
"message": "Username \"admin\" does not exist."
}